The coding interviews at Airbnb are really challenging. The questions are difficult, specific to Airbnb, and cover a wide range of topics.
The good news is that the right preparation can make a big difference. We've analyzed scores of software engineer interview questions reported by Airbnb candidates, in order to determine the most frequently asked types of questions. Below, we've provided a curated list of real example questions, including free solutions.
In addition, you'll find preparation tips and links to the best resources, so that you can prepare more strategically and maximize your chances of landing that software engineer job at Airbnb.
Here's an overview of what we'll cover:
Click here to practice 1-on-1 with a SWE ex-interviewer
1. Interview process and timeline
What's the Airbnb software engineer interview process and timeline? It typically takes 2-5 weeks (according to Glassdoor data), but could take as long as 8+ weeks in some cases. Below are the usual steps in the process:
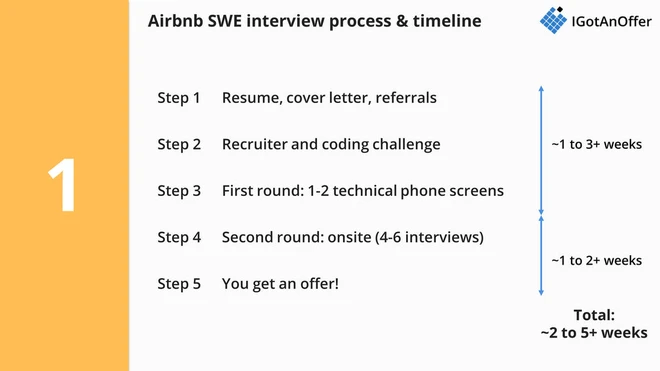
1.1.1 Resume, cover letter, referrals
Step one is getting an Airbnb interview in the first place. In this guide we're focusing primarily on the interviews, so we'll keep this portion brief. You’ll need a quality resume and cover letter that are tailored to SWE positions, and to Airbnb more specifically.
Click here to see our free guide to optimizing your tech resume (with examples).
Once you have your documents together you can submit your application. And, if possible, it can be helpful to get an employee or contact at Airbnb to refer you to the recruiting team internally.
1.1.2 Recruiter and coding challenge
Once your application is approved by Airbnb, then you'll hear from a recruiter (usually through email).
When the recruiter reaches out to you, they'll often ask you to complete a HackerRank coding challenge. Not all candidates are asked to do this, but it's pretty typical. The challenge itself is about 1-hour long, and there will be several test cases you need to pass.
If you'd like to learn more about HackerRank, you can familiarize yourself with some of the challenges that are publicly posted on their website.
1.1.3 First round: 1-2 technical phone screens
If things go well with the recruiter and HackerRank challenge, then you'll move on to the next step of the process.
You'll face 1-2 technical phone screens with a peer or a potential manager at Airbnb. They'll ask you typical data structure and algorithm questions which you'll have to solve in a text editor.
Refer to the section on example coding questions later in this guide to get a better idea of the questions that you'll encounter during your Airbnb interviews.
1.1.4 Second round: onsite (4-6 interviews)
If you crack the first round, the next step is to spend a day at one of Airbnb's offices and to participate in 4-6 onsite interviews.
You can expect about 2 of your onsite interviews to focus on coding questions (i.e. data structure and algorithm questions).
You'll also typically have 2-3 behavioral interviews during your onsite visit. During your behavioral interviews, you'll get a mix of questions about your past experience, and your "fit" with the company culture.
Airbnb puts a large emphasis on these types of behavioral questions, so you'll want to make sure you're well prepared for questions like "Tell me about a difficult project you worked on" or "Why Airbnb?". It's also a good idea to familiarize yourself with Airbnb's mission and core values, which you can read about on their website.
In addition to your coding and behavioral interviews, you may also encounter a system design interview during your onsites. All candidates are expected to do extremely well in coding and behavioral questions, but if you're applying for a relatively junior position, then the bar will be lower on system design questions than for mid-level or senior engineers. You can learn more about system design questions later in this guide.
As a final note, onsite visits will typically be a pretty long day, so it will probably include lunch.
1.1.5 You get an offer!
After your onsite interviews, your job as a candidate is finished. At that point, you'll just need to wait to hear back from Airbnb, to hopefully receive your job offer.
2. Example questions
The types of questions you'll face during your Airbnb interviews can be broken down into 3 broad categories:
- Coding questions
- System design questions
- Behavioral questions
In order to land an offer at Airbnb, you'll want to be well prepared for each of these types of questions. To help you get ready, we've put together a list of interview questions from each category for you to practice with.
2.1 Coding questions
Let's start with coding questions. Below we've compiled a list of coding interview questions. These questions are from Airbnb interview reports posted on Glassdoor, but for each question we've modified the phrasing of the question to match the closest Leetcode problem. We have also linked to a related free solution on Leetcode for each question.
Before you dive into Airbnb's coding questions, there's something else you'll want to know. At some tech companies, you can use general or "quasi-code" for your coding interview questions, where you don't actually have to run your code. But at Airbnb, you'll have to write code that will actually run and also pass test cases.
Finally, we recommend reading this guide on how to answer coding interview questions and practicing with this list of coding interview examples in addition to those listed below.
Example coding questions asked by Airbnb
- "Given an array of distinct integers candidates and a target integer target, return a list of all unique combinations of candidates where the chosen numbers sum to target." (Solution)
- "We are given an elevation map, heights[i] representing the height of the terrain at that index. The width at each index is 1. After V units of water fall at index K, how much water is at each index?" (Solution)
- "You are given some lists of
regions
where the first region of each list includes all other regions in that list...Given two regions region1, region2, find out the smallest region that contains both of them." (Solution) - "You are asked to design a file system that allows you to create new paths and associate them with different values." (Solution)
- "We have n jobs, where every job is scheduled to be done from startTime[i] to endTime[i], obtaining a profit of profit[i]. You're given the startTime, endTime and profit arrays, you need to output the maximum profit you can take such that there are no 2 jobs in the subset with overlapping time range." (Solution)
- "Design and implement an iterator to flatten a 2d vector. It should support the following operations: next and hasNext." (Solution)
- "Given a list of unique words, return all the pairs of the distinct indices (i, j) in the given list, so that the concatenation of the two words words[i] + words[j] is a palindrome." (Solution)
- "You are given coins of different denominations and a total amount of money amount. Write a function to compute the fewest number of coins that you need to make up that amount. If that amount of money cannot be made up by any combination of the coins, return -1." (Solution)
- "Given a puzzle board, return the least number of moves required so that the state of the board is solved. If it is impossible for the state of the board to be solved, return -1." (Solution)
- "There are n cities connected by m flights. Each flight starts from city u and arrives at v with a price w. Now given all the cities and flights, together with starting city src and the destination dst, your task is to find the cheapest price from src to dst with up to k stops. If there is no such route, output -1." (Solution)
2.2 System design questions
In addition to coding questions, you may also face system design questions during your Airbnb interviews. The coding questions we've covered above usually have a single optimal solution. But the system design questions you'll be asked are typically more open-ended and feel more like a discussion.
This is the part of the interview where you want to show that you can both be creative and structured at the same time. In most cases, your interviewer will adapt the question to your background. For instance, if you've worked on an API product they'll ask you to design an API. However, this is not always the case so you should be ready to design any type of product or system at a high level.
Below you'll find a list of example system design questions. It's difficult to find any publicly available system design questions from Airbnb, so as a substitute we've compiled some common questions from Google, Facebook, and Amazon which can be found on Glassdoor.
Although these questions may be a bit different than the exact questions you'd face at Airbnb, the skills and concepts you'll be tested on will be similar, so feel free to practice with these examples.
For more information, we recommend reading this guide on how to answer system design questions and we recommend practicing system design questions here.
Example system design questions
- How would you design Google's database for web indexing
- How would you design a ticketing platform
- How would you design a system that counts the number of clicks on YouTube videos
- How would you design Instagram / Instagram Stories
- How would you design Facebook
- How would you design Twitter's trending topics
- How would you design a warehouse system for Amazon.com
- How would you design Dropbox
- How would you design a real time ranking system for Fortnite
2.3 Behavioral questions
Finally, you'll be asked behavioral interview questions during your Airbnb interviews. Airbnb tends to emphasize candidate "fit" during their behavioral interviews. So, as you prepare, you'll want to get familiar with the company's culture. In addition, you'll need to know the company's mission and values and be able to articulate how you embody them.
Below is a list of behavioral interview questions that were asked at Airbnb according to data from Glassdoor. As a note, we have modified some of the questions below to make them more clear, concise, and grammatically accurate. If you can consistently answer the questions below, that will be a great start to your behavioral interview question preparation for Airbnb.
Example behavioral questions asked at Airbnb
- What are Airbnb's core values and how do they apply to you?
- What does "belong anywhere" mean to you?
- Tell me about a time you've been a good host
- Tell me about a past project and the impact you had
- What have you built?
- What is the most challenging project you've worked on in the last 6 months?
- How do you give back to the community?
- Tell me about a time you had to give someone terrible news
- What do you like about Airbnb?
- What is one thing you'd like to remove from Airbnb?
3. How to prepare
Now that you know the types of questions to expect, let's focus on how to prepare. Here are the four most important things you can do to prepare for your Airbnb software engineer interviews.
3.1 Learn about Airbnb's culture and values
Most candidates fail to do this, but before investing a ton of time preparing for Airbnb interviews, you should take some time to make sure it's actually the right company for you. This is especially important for Airbnb, given how heavily they emphasize culture fit.
If you know engineers who work at Airbnb (or used to), talk to them to understand what the culture is like. In addition, we would also recommend reading the following resources.
- Airbnb's mission and values (from Airbnb)
- Airbnb strategy teardown (from CB Insights)
- What Seven Years at Airbnb Taught Me About Building a Business (from Medium)
3.2 Practice by yourself
As mentioned above, Airbnb asks three types of questions during their interviews: coding, system design, and behavioral. The first step of your preparation should be to brush up on these different types of questions and to practice answering them by yourself.
3.2.1 Coding interview preparation
Airbnb hasn't published specific guidance on their coding interview preparation. However, there are some really helpful resources that have been published by other large tech companies.
For example, we feel that the Microsoft video below provides good general guidance for preparing for coding interviews. Just keep in mind that at Airbnb you'll usually be doing your coding interviews directly in a text editor on a computer (rather than on a whiteboard).
Here is a summary of the STAR approach recommended by Microsoft in the video above:
- Step 1: Situation, make sure you understand the problem correctly
- Write down the problem provided by the interviewer
- Ask clarification questions
- Agree on inputs and outputs
- Step 2: Task, outline how you are going to solve the problem
- Talk through your ideas for solving the problem out loud
- Start with a simple case and work toward a solution, without coding
- Think about different test cases which you'll use for testing later
- Step 3: Action, write clean code to solve the problem
- Start writing clean code with clear variable and function names
- Modularize your code with helper functions
- Check with your interviewer before using a library
- Bugs and forgotten variables are ok as long as you catch them early
- Step 4: Result, test your solution and suggest improvements
- Run through the different test cases you've developed in step 2
- Incorporate any feedback from the interviewer into your approach
- Analyze the time and space complexity of your code and suggest improvements
For the rest of your coding prep, we recommend using our coding interview prep article as your one-stop-shop to guide you through your preparation process.
3.2.2 System design interview preparation
For system design interviews, we think you'll find the approach outlined in the below video from Amazon to be quite helpful. Again, this advice is coming from another company (i.e. Amazon not Airbnb), but the overall skills and approach should be transferable.
Here is a summary of what you should do:
- Step 1: Ask clarification questions
- Understand the goal of the system (e.g. sell ebooks)
- Establish the scope of the exercise (e.g. end-to-end experience, or just API?)
- Gather scale and performance requirements (e.g. 500 transactions per second)
- Talk through any assumptions you're making out loud
- Step 2: Design at a high-level, then drill-down
- Lay out the high-level components (e.g. front-end, web servers, database)
- Drill down and design each component (e.g. front-end first)
- Start with the components you're most comfortable with (e.g. front-end if you're a front-end engineer)
- Work with your interviewer to provide the right level of detail
- Step 3: Bring it all together
- Refer back to the requirements to make sure your approach meets them
- Discuss any tradeoffs in the decisions you've made
- Summarize how the system would work end-to-end
We'd also recommend studying our system design interview prep guide and learning how to answer system design interview questions. These guides cover a step-by-step method for answering system design questions, and they provide several example questions with solutions.
3.2.3 Behavioral interview question preparation
For behavioral interviews, we recommend learning our step-by-step method to answer this type of question and then practicing the most common software engineer behavioral interview questions.
Finally, a great way to practice coding, system design, and behavioral questions, is to interview yourself out loud. This may sound strange, but it can significantly improve the way you communicate your answers during a real interview. Play the role of both the candidate and the interviewer, asking questions and answering them, just like two people would in an interview.
However, by yourself, you can’t simulate thinking on your feet or the pressure of performing in front of a stranger. Plus, there are no unexpected follow-up questions and no feedback.
That’s why many candidates try to practice with friends or peers.
3.3 Practice with peers
If you have friends or peers who can do mock interviews with you, that's an option worth trying. It’s free, but be warned, you may come up against the following problems:
- It’s hard to know if the feedback you get is accurate
- They’re unlikely to have insider knowledge of interviews at your target company
- On peer platforms, people often waste your time by not showing up
For those reasons, many candidates skip peer mock interviews and go straight to mock interviews with an expert.
3.4 Practice with experienced SWE interviewers
In our experience, practicing real interviews with experts who can give you company-specific feedback makes a huge difference.
Find an Airbnb software engineer interview coach so you can:
- Test yourself under real interview conditions
- Get accurate feedback from a real expert
- Build your confidence
- Get company-specific insights
- Learn how to tell the right stories, better.
- Save time by focusing your preparation
Landing a job at a big tech company often results in a $50,000 per year or more increase in total compensation. In our experience, three or four coaching sessions worth ~$500 make a significant difference in your ability to land the job. That’s an ROI of 100x!